TICKET MACHINE
Berikut ini adalah aplikasi mesin tiket sederhana menggunakan bahasa pemrograman java
MAIN SOURCE CODE
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
| //Main
import java.util.Scanner;
public class IntMain
{
public static void main(String args[])
{
Scanner scan= new Scanner(System.in);
int cost,menu;
System.out.println("Masukkan harga tiket \n");
cost=scan.nextInt();
TicketMachine ticket=new TicketMachine(cost);System.out.println("1. Get Price");
System.out.println("2. Get Balance");
System.out.println("3. Insert Money");
System.out.println("4. Print Ticket");
menu=scan.nextInt();
switch(menu)
{
case 1:
cost=ticket.getPrice();
System.out.println(cost);
break;
case 2:
ticket.getBalance();
break;
case 3:
int money=scan.nextInt();
ticket.insertMoney(money);
break;
case 4:
ticket.printTicket();
break;
}
}
}
|
TICKET MACHINE SC
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
| public class TicketMachine
{
// The price of a ticket from this machine.
private int price;
// The amount of money entered by a customer so far.
private int balance;
// The total amount of money collected by this machine.
private int total;
/**
* Create a machine that issues tickets of the given price.
* Note that the price must be greater than zero, and there
* are no checks to ensure this.
*/
public TicketMachine(int ticketCost)
{
price = ticketCost;
balance = 0;
total = 0;
}
/**
* Return the price of a ticket.
*/
public int getPrice()
{
return price;
}
/**
* Return the amount of money already inserted for the
* next ticket.
*/
public int getBalance()
{
return balance;
}
/**
* Receive an amount of money in cents from a customer.
*/
public void insertMoney(int amount)
{
balance = balance + amount;
}
/**
* Print a ticket.
* Update the total collected and
* reduce the balance to zero.
*/
public void printTicket()
{
// Simulate the printing of a ticket.
System.out.println("##################");
System.out.println("# The BlueJ Line");
System.out.println("# Ticket");
System.out.println("# " + price + " cents.");
System.out.println("##################");
System.out.println();
// Update the total collected with the balance.
total = total + balance;
// Clear the balance.
balance = 0;
}
}
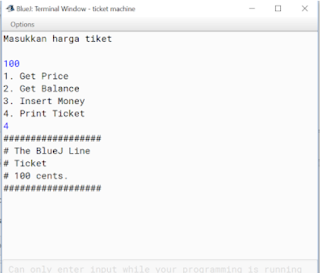
|
Comments
Post a Comment